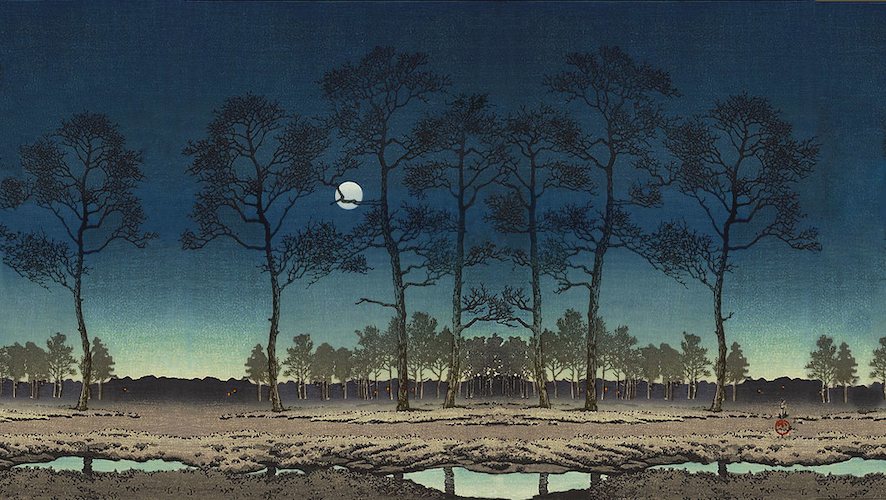
Easiest way to format a date in Eleventy
April 13th, 2025When creating this website, I wanted to show the date of each post I made, as you can see on my articles page.
To add a date that a post was written, you would just add date: yyyy-mm-dd in the front matter, and later display it with post.data.date.
This works, however, the displayed date would look like this:
Sun Apr 13 2025 02:00:00 GMT+0200 (Central European Summer Time)
Instead of something like this:
April 13th, 2025
We can achieve this by using eleveenty filters. You can read more about them here.
Step 1: Import Luxon
Luxon comes preinstalled with Eleventy, so you just need to add this to the top of your .eleventy.js config file:
const { DateTime } = require("luxon");
Step 2: Add a custom filter for formatting dates
Add the following code to .eleventy.js config file:
module.exports = function (eleventyConfig) {
// other code...
eleventyConfig.addFilter("readableDate", (dateObj) => {
let dt;
if (typeof dateObj === "string") {
dt = DateTime.fromISO(dateObj);
} else if (dateObj instanceof Date) {
dt = DateTime.fromJSDate(dateObj);
} else {
throw new Error("Invalid date format");
}
const day = dt.day;
const ordinal = getOrdinal(day);
// LLLL - Full month name (e.g., April)
// d - Day of the month
// yyyy - Four-digit year
return dt.toFormat(`LLLL d'${ordinal}', yyyy`);
});
// Helper function to calculate ordinal suffixes
function getOrdinal(day) {
if (day > 3 && day < 21) return "th";
switch (day % 10) {
case 1:
return "st";
case 2:
return "nd";
case 3:
return "rd";
default:
return "th";
}
}
// other code...
};
Now, you have a custom filter that will show a formatted date and it will also check if the date is a string (ISO date string) or a JavaScript Date object, so no errors will pop out. You can always modify this format by changing this line of code:
return dt.toFormat(`LLLL d'${ordinal}', yyyy`);
Or even removing the whole ordinal if you don't like it.
Step 3: Use the filter
The only thing that's left now is to use this filter, and you can do that by just adding the filter name that you declared earlier to the place you're displaying the date, like this:
{{ article.data.date | readableDate }}
That's it! Now you have a nicely formatted date that looks like this: April 13th, 2025.